How We Built an AI-Powered Kitchen Planner (with OpenAI, Weaviate, and FastAPI) — A Complete DIY Guide
Imagine being able to generate a complete kitchen design with the perfect products, layout, and estimated budget — just by entering your kitchen size and layout type. That’s exactly what we built. In this blog, I’ll walk you through the exact process we followed to build an intelligent AI-powered kitchen planner. Whether you're a developer, a CTO exploring generative AI, or a founder looking to build your MVP faster — this is your no-fluff, step-by-step guide.
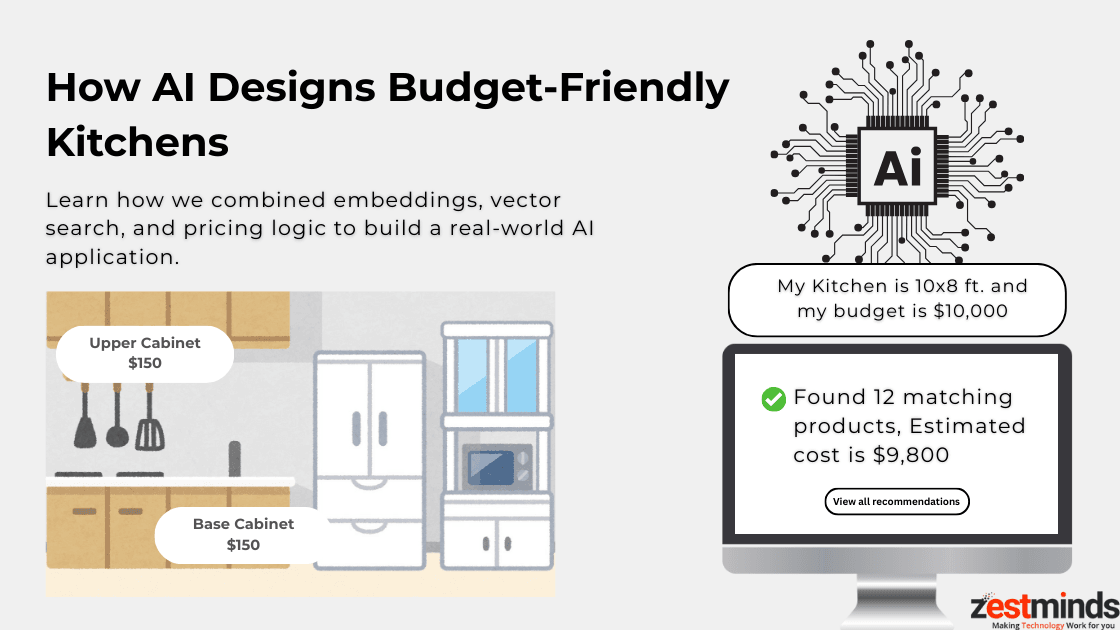
What Are We Building?
We’re creating a system that can:
- Accept kitchen layout type, size, and budget from users
- Analyze historical kitchen solution data
- Recommend product combinations and layout ideas
- Estimate costs — all automatically using OpenAI, Weaviate, and FastAPI
This isn’t just a blog — it’s a developer blueprint for building RAG-based (Retrieval-Augmented Generation) AI apps using real-world unstructured data.
Related Read: What is Retrieval-Augmented Generation (RAG)?
The Problem Statement (and Why It’s So Common)
We had:
- PDF product catalogs
- Proprietary .PRJZ layout files
- CSV price lists
We needed to:
- Extract structured data
- Clean and normalize it
- Store it for querying
- Use embeddings to build similarity search
- Recommend based on matching vectors
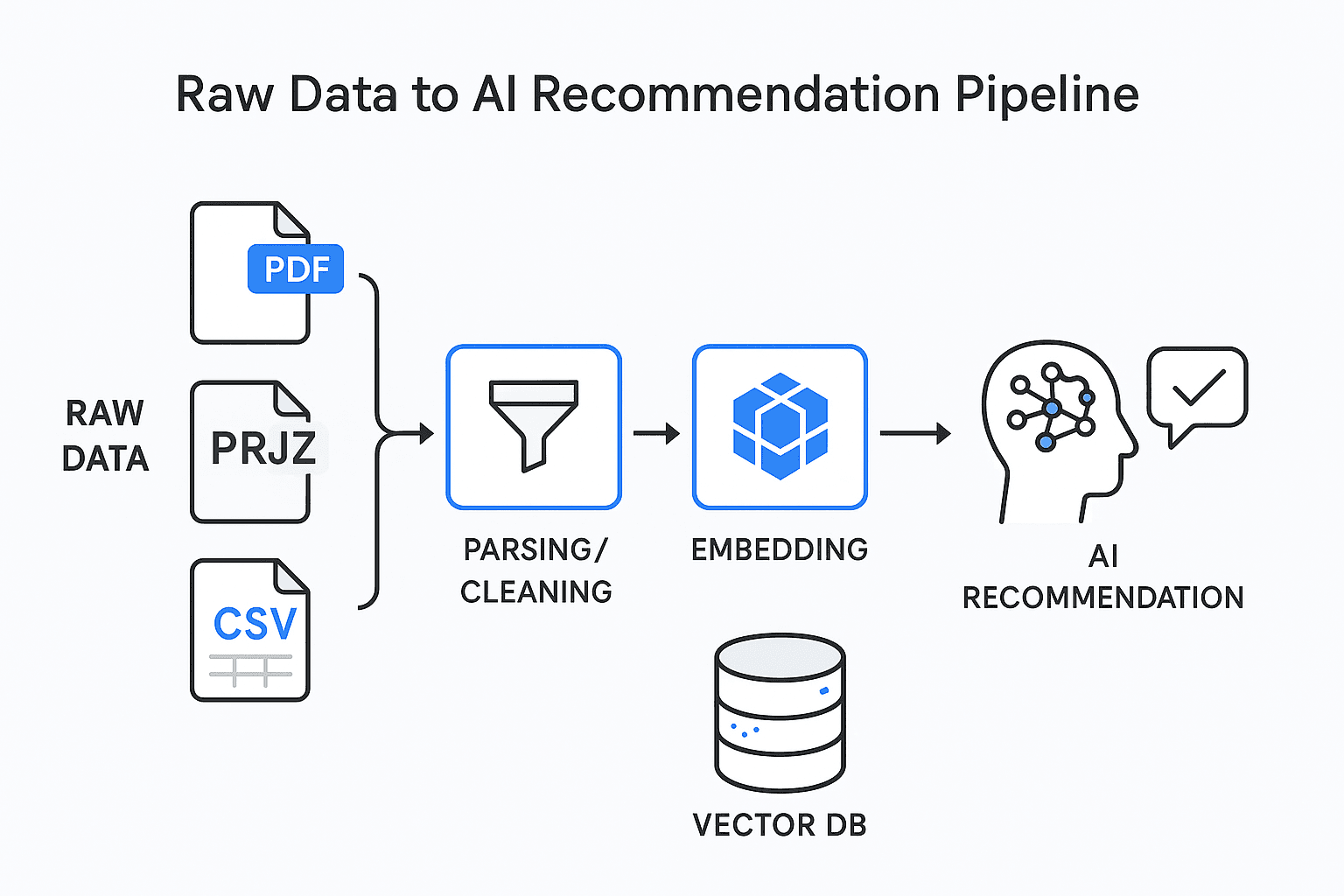
Step 1: Extract Data From PDF, PRJZ, and CSV
Parsing PDF Product Catalogs
We used pdfplumber
to extract product information like name, size, category, and description.
import pdfplumber
with pdfplumber.open('products.pdf') as pdf:
for page in pdf.pages:
text = page.extract_text()
print(text)
Decoding .PRJZ Files
These were proprietary project files. We renamed them to .zip
, extracted contents, and found XML inside!
import zipfile
with zipfile.ZipFile('solution.prjz', 'r') as zip_ref:
zip_ref.extractall("solution_data")
Then parsed the XML:
import xml.etree.ElementTree as ET
tree = ET.parse('solution_data/solution.xml')
root = tree.getroot()
for layout in root.findall('layout'):
print(layout.find('type').text)
Reading the Price List
Easy with Pandas:
import pandas as pd
prices = pd.read_csv("price_list.csv")
Step 2: Clean and Structure the Data
We normalized:
- Product names
- Dimensions
- Category values
And created 3 MySQL tables:
products (id, name, dimensions, category, description)
price_list (product_id, price)
solutions (id, layout_type, width, height, product_ids, total_cost)
Related: How We Build Custom GPT Apps with Pinecone & Streamlit
Step 3: Convert Each Entry Into Natural Language
Because OpenAI doesn’t work with tables, we converted each row into a readable sentence:
f"{name} is a {dimensions} kitchen product used for {category}. Description: {description}"
Solutions were transformed into:
f"A {layout_type} layout with dimensions {width}x{height} uses these products: {product_names}. Estimated cost is {total_cost}."
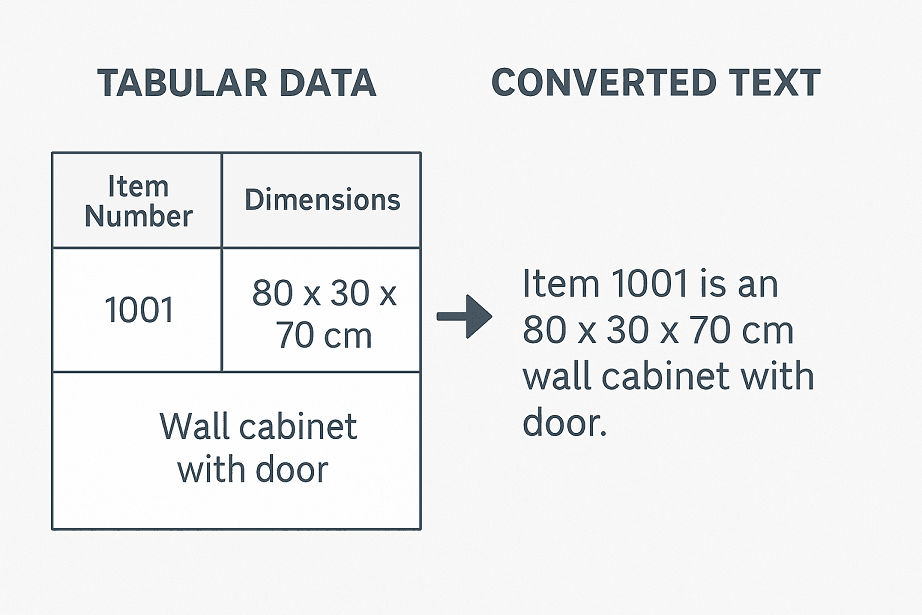
Step 4: Generate Embeddings Using OpenAI
We used text-embedding-3-small from OpenAI.
import openai
openai.api_key = "sk-xxx"
response = openai.Embedding.create(
model="text-embedding-3-small",
input="Oak cabinet, 60x90cm, under-counter storage"
)
vector = response['data'][0]['embedding']
Related: Build AI Chatbots Using OpenAI + Streamlit
Step 5: Set Up Weaviate (Our Vector Search Engine)
Docker Setup
version: '3'
services:
weaviate:
image: semitechnologies/weaviate:latest
ports:
- "8080:8080"
environment:
- QUERY_DEFAULTS_LIMIT=25
- AUTHENTICATION_ANONYMOUS_ACCESS_ENABLED=true
- PERSISTENCE_DATA_PATH=/var/lib/weaviate
- DEFAULT_VECTORIZER_MODULE=none
volumes:
- ./weaviate-data:/var/lib/weaviate
Define Schema
import weaviate
client = weaviate.Client("http://localhost:8080")
client.schema.create_class({
"class": "Product",
"properties": [
{"name": "name", "dataType": ["text"]},
{"name": "description", "dataType": ["text"]},
{"name": "price", "dataType": ["number"]}
]
})
Also Read: OpenAI + Weaviate for Smart Search in Fintech
Step 6: Match User Input With Similar Solutions
Convert User Input to Embedding
query = "Looking for an L-shape kitchen in 10x8 ft space with $10,000 budget"
query_vector = get_embedding(query)
Query Weaviate
results = client.query.get("Solution", ["layout_type", "total_cost", "product_ids"]).with_near_vector({
"vector": query_vector
}).with_limit(3).do()
Then filter by layout type, dimensions, and budget range.
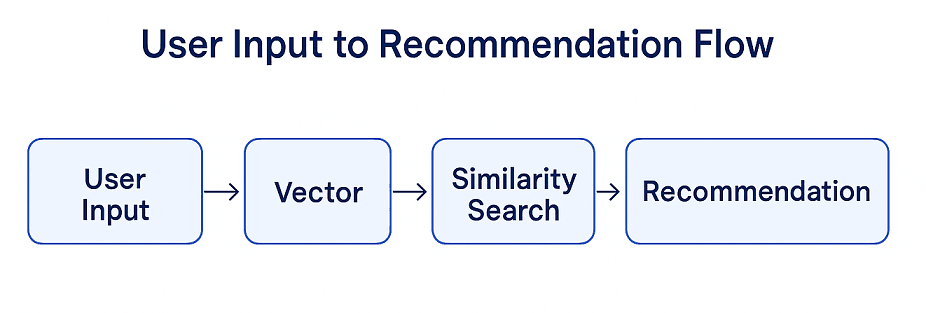
Step 7: Build FastAPI Backend
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class UserInput(BaseModel):
layout_type: str
width: int
height: int
budget: float
@app.post("/recommend")
def recommend(input: UserInput):
# Process & vector search logic
return {
"products": ["Oak Cabinet", "Sink Unit"],
"estimated_cost": "$9,800"
}
Step 8: Frontend UI (Basic Example)
HTML form + fetch example:
<form onsubmit="submitForm()">
<input name="layout_type" placeholder="L-shape">
<input name="width" type="number" placeholder="Width">
<input name="height" type="number" placeholder="Height">
<input name="budget" type="number" placeholder="Budget">
<button>Get Recommendations</button>
</form>
Summary: What You Learned
- How to handle messy PDF/XML/CSV data
- How to use OpenAI embeddings to represent product & layout ideas
- How to store and search vectors in Weaviate
- How to wrap it up in a real-time FastAPI app
This system is flexible, scalable, and a great MVP for any AI assistant use case — not just kitchens.
Related Case Study: HIPAA-Compliant AI Hospital System
Want to Build Something Like This?
We help startups and CTOs build and ship AI-powered MVPs fast.
Want the full source code or architecture diagram? Book a free consult call.
Frequently Asked Questions – AI Kitchen Planner
Can this system recommend kitchens within any budget?
Yes, the AI model uses vector similarity search and pricing logic to evaluate whether a valid kitchen solution can be created within a user’s specified budget range, with a ±10% flexibility margin.
What is a PRJZ file and how do you process it?
A .PRJZ file is a proprietary format often used by kitchen design software. We attempt to extract structured data by unzipping or converting it, or guide the client to export it as CSV or XML for ingestion.
Why use OpenAI embeddings instead of keyword search?
OpenAI embeddings allow the system to search based on semantic meaning, not just keywords. This helps match similar past kitchen layouts, even if the product names or descriptions are worded differently.
Can I replace OpenAI with a local model later?
Yes, the system architecture is modular. You can swap OpenAI with open-source LLMs like Mistral or LLaMA and use a local embedding model such as sentence-transformers
or HuggingFace models.
How does the system ensure only eligible products are used?
The recommendation engine filters out any product not present in the client’s provided price list. Only items with valid, listed prices are included in the final solution.
Can I replace Weaviate with Pinecone or Qdrant?
Yes! The vector DB is modular.
Will this scale to thousands of users?
Yes, with proper caching, batching, and async APIs.
Can I embed this in a mobile app?
Absolutely. Build a React Native or Flutter frontend and connect it to the same backend.
How secure is this system?
Use environment variables, add auth, and self-host Weaviate if needed.
What’s the hardest part?
Honestly? Converting messy files into clean, structured data.
Enjoyed this tutorial?
Check out more developer-focused content:
The Future of AI Chatbot Development
Generative AI Applications Across Industries
Secure Auth with Supabase + Next.js
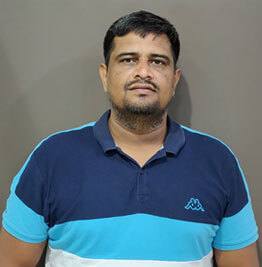
Shivam Sharma
About the Author
With over 13 years of experience in software development, I am the Founder, Director, and CTO of Zestminds, an IT agency specializing in custom software solutions, AI innovation, and digital transformation. I lead a team of skilled engineers, helping businesses streamline processes, optimize performance, and achieve growth through scalable web and mobile applications, AI integration, and automation.